RadioGroup
Om komponenten
RadioGroup lar brukeren enkelt skanne og sammenligne alternativer for å ta ett valg.
Egnet til:
- Handlinger der brukeren skal velge kun ett alternativ fra en liste på fem eller færre.
Uegnet til:
- Der bruker kan krysse av og på for ett alternativ, eller der flere valg i listen er tillatt. Se Checkbox eller Select.

Varianter
Uncontrolled
En uncontrolled RadioGroup med tre valg.
<RadioGroup> <Radio value="ordinary">Ordinær pris</Radio> <Radio value="bostart" description="OBOS Bostart gjør at du kan kjøpe en helt ny bolig til en lavere pris enn ordinær markedspris." > OBOS Bostart </Radio> <Radio value="deleie" description="Med OBOS Deleie kan du kjøpe halve boligen, eller mer, og bo i hele." > OBOS Deleie </Radio> </RadioGroup>
En uncontrolled RadioGroup med default valg.
<RadioGroup defaultValue="deleie" > <Radio value="ordinary">Ordinær pris</Radio> <Radio value="bostart" description="OBOS Bostart gjør at du kan kjøpe en helt ny bolig til en lavere pris enn ordinær markedspris." > OBOS Bostart </Radio> <Radio value="deleie" description="Med OBOS Deleie kan du kjøpe halve boligen, eller mer, og bo i hele." > OBOS Deleie </Radio> </RadioGroup>
En uncontrolled RadioGroup med beskrivelse.
<RadioGroup description="Kontakt oss for mer utfyllende informasjon om de forskjellige alternativene" > <Radio value="ordinary">Ordinær pris</Radio> <Radio value="bostart" description="OBOS Bostart gjør at du kan kjøpe en helt ny bolig til en lavere pris enn ordinær markedspris." > OBOS Bostart </Radio> <Radio value="deleie" description="Med OBOS Deleie kan du kjøpe halve boligen, eller mer, og bo i hele." > OBOS Deleie </Radio> </RadioGroup>
Validering
En påkrevd RadioGroup.
<form onSubmit={(e) => e.preventDefault()} className="grid gap-6" > <RadioGroup isRequired> <Radio value="ordinary">Ordinær pris</Radio> <Radio value="bostart" description="OBOS Bostart gjør at du kan kjøpe en helt ny bolig til en lavere pris enn ordinær markedspris." > OBOS Bostart </Radio> <Radio value="deleie" description="Med OBOS Deleie kan du kjøpe halve boligen, eller mer, og bo i hele." > OBOS Deleie </Radio> </RadioGroup> <Button type="submit" className="w-fit">Send inn</Button> </form>
En invalid RadioGroup.
<RadioGroup isInvalid > <Radio value="ordinary">Ordinær pris</Radio> <Radio value="bostart" description="OBOS Bostart gjør at du kan kjøpe en helt ny bolig til en lavere pris enn ordinær markedspris." > OBOS Bostart </Radio> <Radio value="deleie" description="Med OBOS Deleie kan du kjøpe halve boligen, eller mer, og bo i hele." > OBOS Deleie </Radio> </RadioGroup>
Controlled
En controlled CheckboxGroup
function(){ const [value, setValue] = React.useState('deleie'); return <RadioGroup label="Jeg er interessert i" value={value} onChange={(newValue) => setValue(newValue)} > <Radio value="ordinary">Ordinær pris</Radio> <Radio value="bostart" description="OBOS Bostart gjør at du kan kjøpe en helt ny bolig til en lavere pris enn ordinær markedspris." > OBOS Bostart </Radio> <Radio value="deleie" description="Med OBOS Deleie kan du kjøpe halve boligen, eller mer, og bo i hele." > OBOS Deleie </Radio> </CheckboxGroup>}

Retningslinjer
Ikke bruk radioknapper om brukeren skal kunne velge flere valg
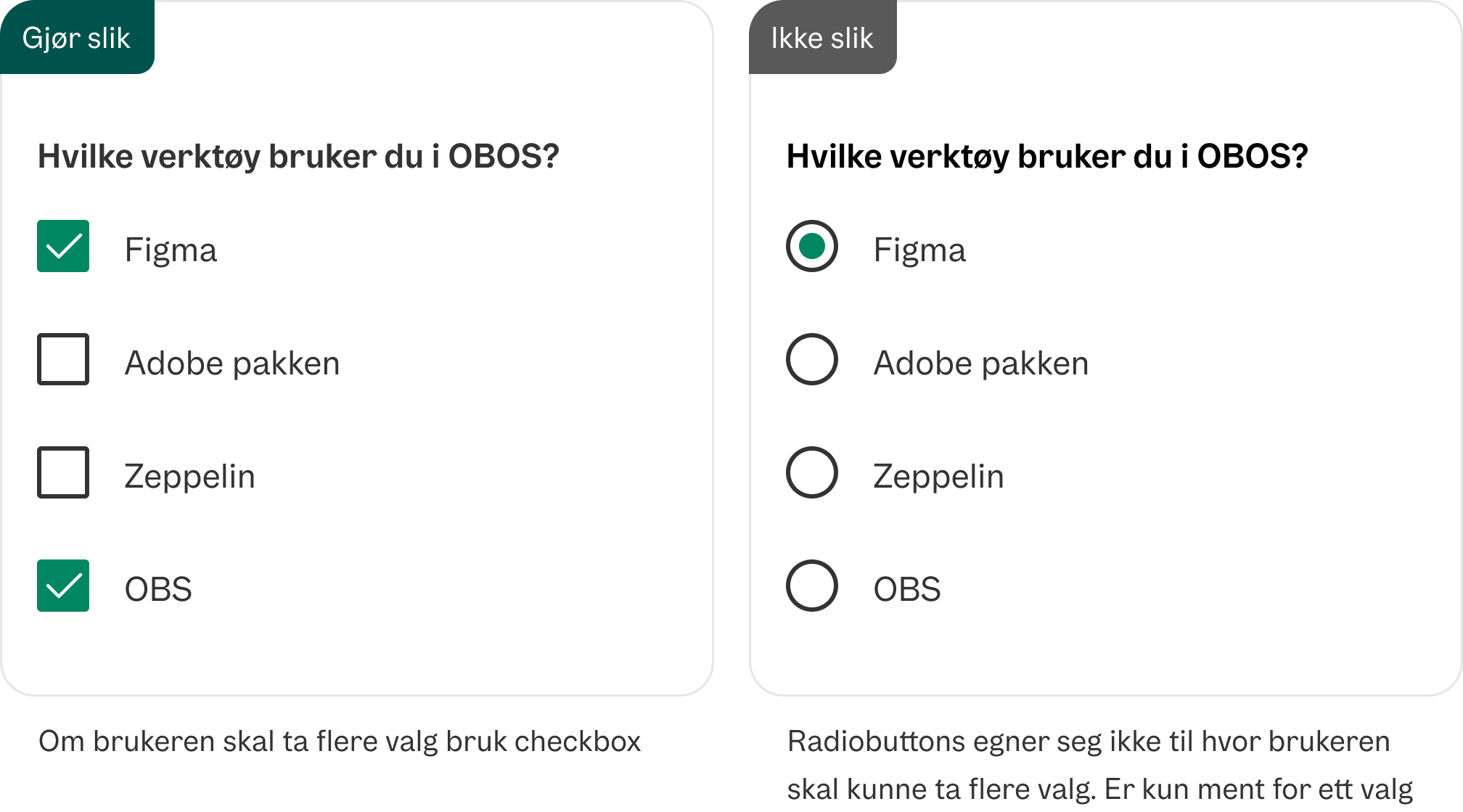
Ikke bruk RadioButton dersom du har mange valg
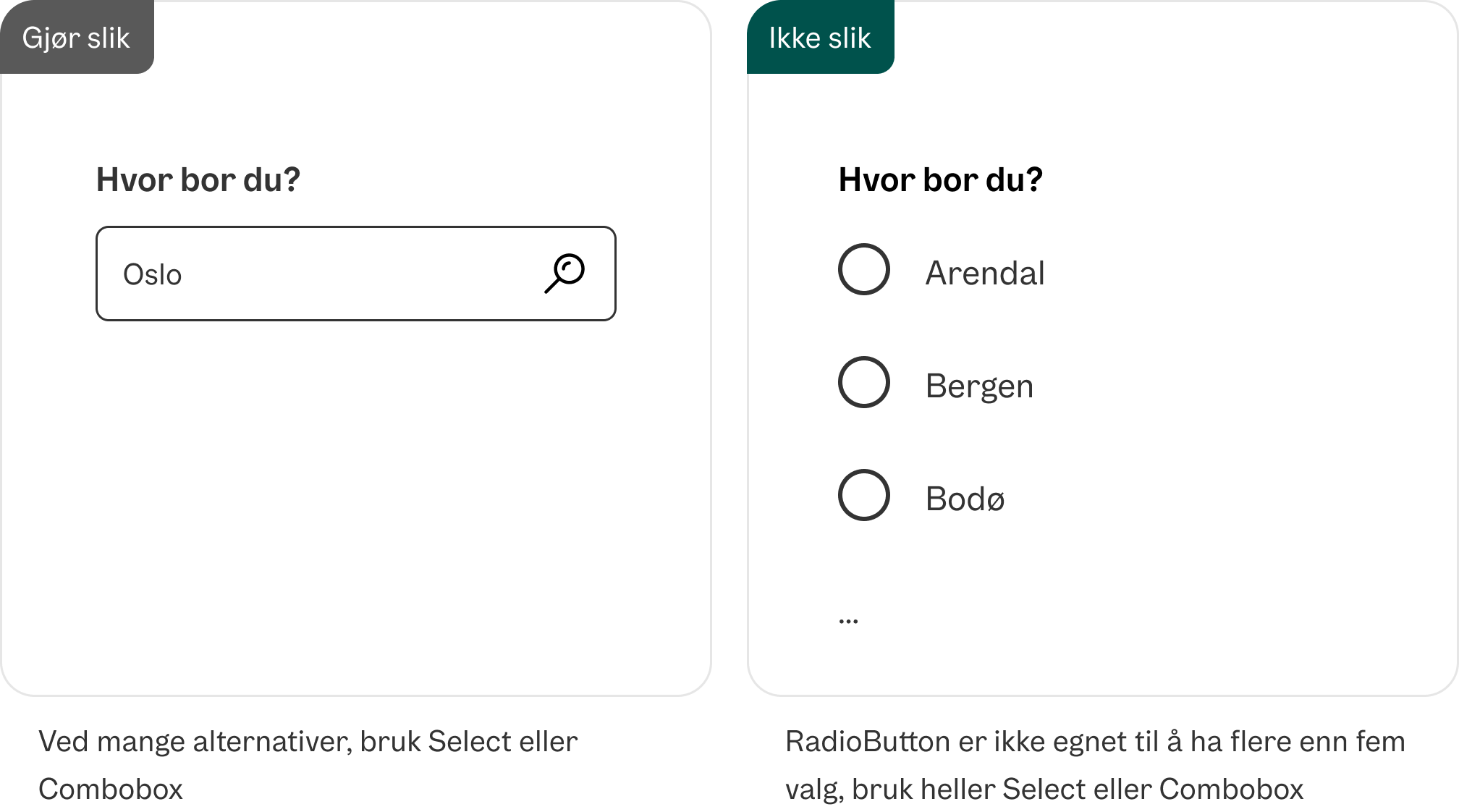

Formatering
Anatomi
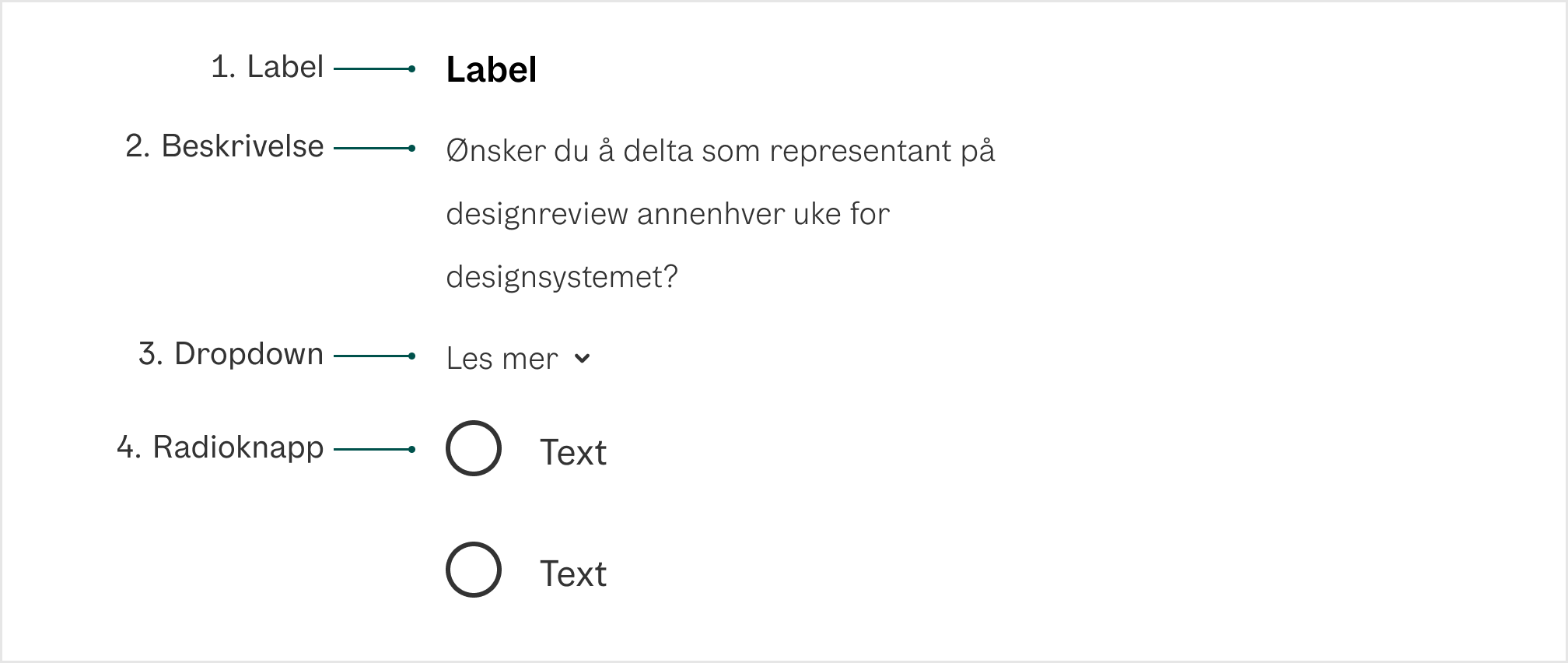
1. Label Forteller brukeren hva som skal velges
2. Beskrivelse (valgfri) Hjelpetekst med mer informasjon eller gir kontekst til brukeren.
3. Dropdown (valgfri) Ekspanderende felt som skal supplere hjelpeteksten med mer informasjon. Viktig informasjon skal ikke legges her.
4. RadioButton En radioknapp som indikerer riktig tilstand. Som standard er det ikke valgt.

Universell utforming
Husk på
- Alle radioknappene må ha klare, konsise og unike labels. WCAG 1.3.1 og 2.4.6
- Unngå standardvalg: Forhåndsvalgte valg anses som en villedende praksis for alle brukere. Gå for at brukeren selv skal kunne ta et aktivt valg når du bruker radioknapper.
- Alle tastaturinteraksjoner og ARIA-etiketter må oppfylle tilgjengelighetskriterier (WAI-ARIA Checkbox Authoring Practices - Gjelder også for Radiobuttons)
Sjekkliste
✓ Alle radioknappene har en klar, kortfattet og unik label
✓ Ingen av radioknappene har et satt standardvalg
✓ Brukeren kan tabbe seg gjennom radioknappene, og velge en av dem ved å trykke space
Les mer
WCAG 1.3.1 Info and Relationships (A)
Props
RadioGroup
Prop | Description | Default |
---|---|---|
className? | Additional CSS className for the element. | - |
description? | Help text for the form control. | - |
errorMessage? | Error message for the form control. Automatically sets `isInvalid` to true | - |
label? | Label for the form control. | - |
style? | Additional style properties for the element. | - |
ref? | Ref for the element. | - |
onFocus? | Handler that is called when the element receives focus. | - |
onBlur? | Handler that is called when the element loses focus. | - |
onFocusChange? | Handler that is called when the element's focus status changes. | - |
id? | The element's unique identifier. See [MDN](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/id). | - |
aria-label? | Defines a string value that labels the current element. | - |
aria-labelledby? | Identifies the element (or elements) that labels the current element. | - |
aria-describedby? | Identifies the element (or elements) that describes the object. | - |
aria-details? | Identifies the element (or elements) that provide a detailed, extended description for the object. | - |
name? | The name of the input element, used when submitting an HTML form. See [MDN](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input#htmlattrdefname). | - |
value? | The current value (controlled). | - |
validationBehavior? | Whether to use native HTML form validation to prevent form submission when the value is missing or invalid, or mark the field as required or invalid via ARIA. | 'native' |
onChange? | Handler that is called when the value changes. | - |
isRequired? | Whether user input is required on the input before form submission. | - |
isInvalid? | Whether the input value is invalid. | - |
validate? | A function that returns an error message if a given value is invalid. Validation errors are displayed to the user when the form is submitted if `validationBehavior="native"`. For realtime validation, use the `isInvalid` prop instead. | - |
aria-errormessage? | Identifies the element that provides an error message for the object. | - |
slot? | A slot name for the component. Slots allow the component to receive props from a parent component. An explicit `null` value indicates that the local props completely override all props received from a parent. | - |
defaultValue? | The default value (uncontrolled). | - |
Radio
Prop | Description | Default |
---|---|---|
className? | Additional CSS className for the element. The CSS [className](https://developer.mozilla.org/en-US/docs/Web/API/Element/className) for the element. A function may be provided to compute the class based on component state. | - |
description? | Help text for the form control. | - |
style? | Additional style properties for the element. | - |
ref? | Ref for the element. | - |
autoFocus? | Whether the element should receive focus on render. | - |
onFocus? | Handler that is called when the element receives focus. | - |
onBlur? | Handler that is called when the element loses focus. | - |
onFocusChange? | Handler that is called when the element's focus status changes. | - |
onKeyDown? | Handler that is called when a key is pressed. | - |
onKeyUp? | Handler that is called when a key is released. | - |
id? | The element's unique identifier. See [MDN](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/id). | - |
aria-label? | Defines a string value that labels the current element. | - |
aria-labelledby? | Identifies the element (or elements) that labels the current element. | - |
aria-describedby? | Identifies the element (or elements) that describes the object. | - |
aria-details? | Identifies the element (or elements) that provide a detailed, extended description for the object. | - |
value | The value of the radio button, used when submitting an HTML form. See [MDN](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input/radio#Value). | - |
inputRef? | A ref for the HTML input element. | - |
onHoverStart? | Handler that is called when a hover interaction starts. | - |
onHoverEnd? | Handler that is called when a hover interaction ends. | - |
onHoverChange? | Handler that is called when the hover state changes. | - |
slot? | A slot name for the component. Slots allow the component to receive props from a parent component. An explicit `null` value indicates that the local props completely override all props received from a parent. | - |